Logistic Regression Python Code
In data science, logistic regression is an essential method, especially when dealing with categorization issues. It is frequently used to estimate probability and forecast binary outcomes. This article will explore the nuances of logistic regression, including how to build it in Python and use it in real-world scenarios.
1. Introduction to Logistic Regression
What is logistic regression?
One statistical technique for binary classification problems is logistic regression. Logistic regression forecasts the likelihood that an instance will belong to a specific class, as opposed to linear regression, which predicts continuous values.
Why is logistic regression important in data science?
Logistic regression’s interpretability and simplicity make it an essential tool in data research. It’s a standard technique for forecasting and categorical data analysis in a number of industries, including marketing, finance, healthcare, and more.
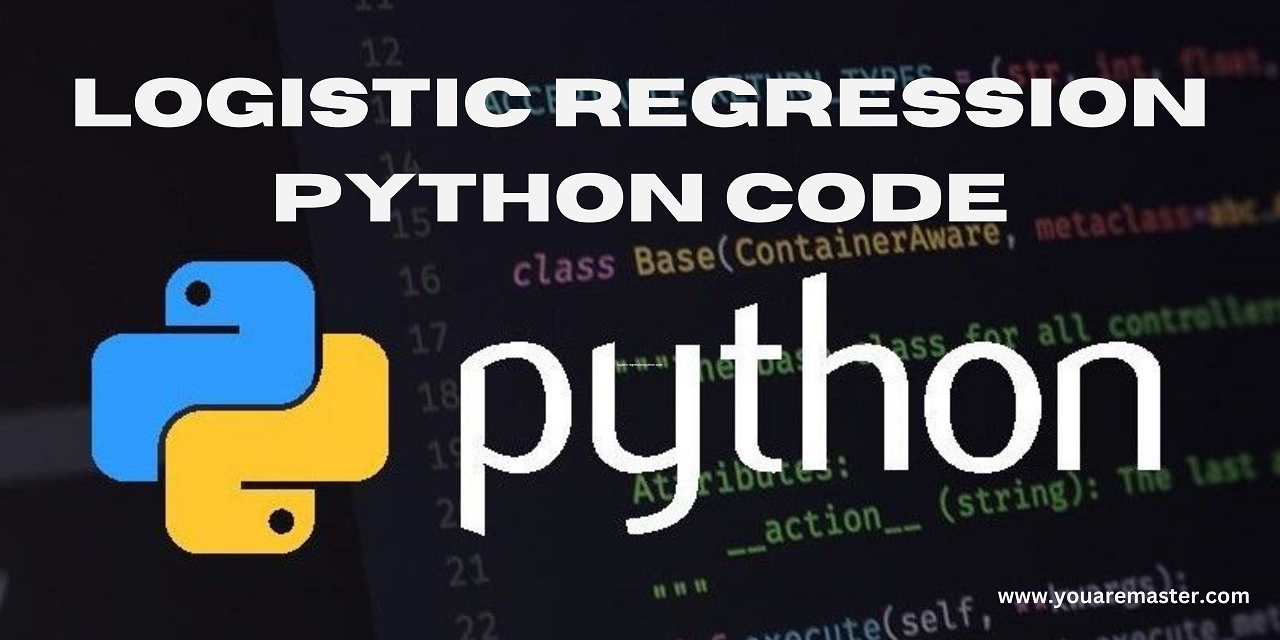
2. Understanding the Logistic Regression Algorithm
Basic concepts of logistic regression
Fundamentally, logistic regression uses the logistic function, also referred to as the sigmoid function, to describe the likelihood that a given input belongs to a particular class. This function may be used for binary classification as it transfers any real-valued integer to the interval [0, 1].
Mathematical formulation
The logistic regression model computes the probability 𝑃(𝑦=1∣𝑋) of an instance belonging to class 1 given the input features 𝑋. It’s represented by the sigmoid function:
𝑃(𝑦=1∣𝑋)=11+𝑒−(𝛽0+𝛽1𝑋1+…+𝛽𝑛𝑋𝑛)
Where:
- 𝛽0,𝛽1,…,𝛽𝑛 are the model coefficients.
- 𝑋1,𝑋2,…,𝑋𝑛 are the input features.
3. Applications of Logistic Regression Python
Classification problems
For binary classification problems like spam email detection, illness diagnosis, and sentiment analysis, logistic regression is frequently utilized.
Predicting probabilities
In addition to classifying data, logistic regression may be used to determine the likelihood that a given instance falls into a certain class, which can yield important information for decision-making.
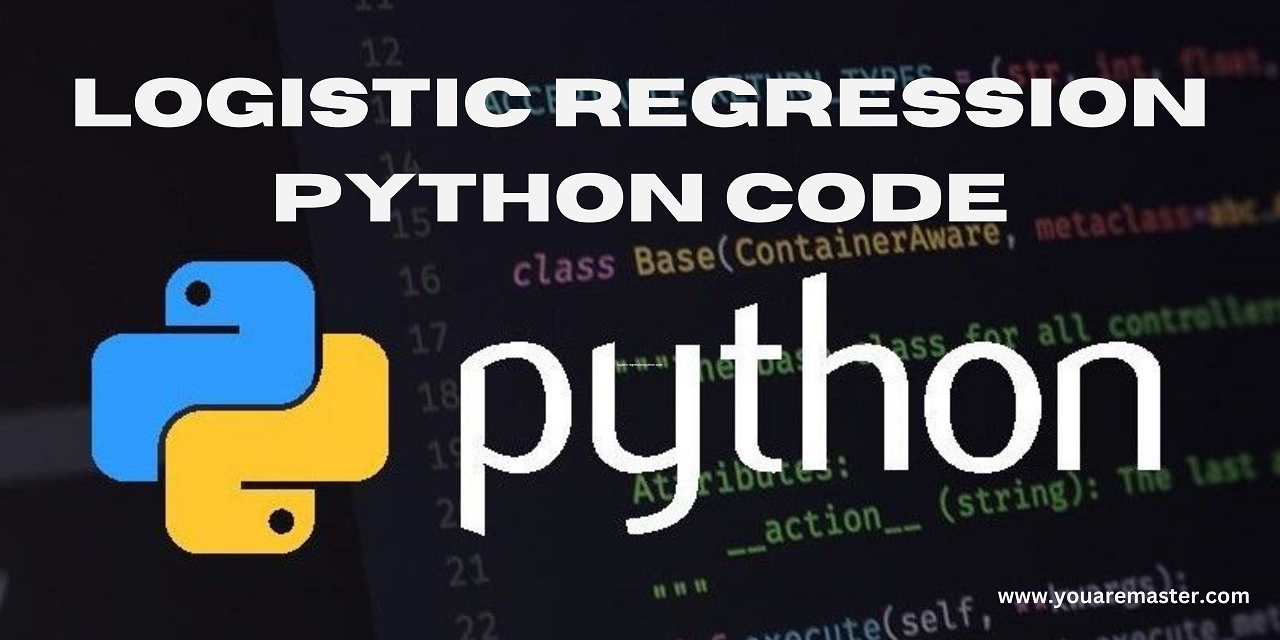
4. Implementing Logistic Regression Python
Setting up the environment
Make sure you have Python installed along with necessary libraries like NumPy, Pandas, and Scikit-learn before beginning implementation.
Importing necessary libraries
Import the necessary libraries, such as Pandas, NumPy, Matplotlib, and Scikit-learn, for data processing, visualization, and model creation.
Data preprocessing
Divide the data into training and testing sets, handle missing values, and encode categorical variables to prepare the dataset.
Model training
Utilising the training data, train the logistic regression model, then assess its performance with suitable measures like accuracy, precision, recall, and F1-score.
Model evaluation
Evaluate the model’s performance using the testing data to make sure it can generalize and, if needed, pinpoint areas that need improvement.
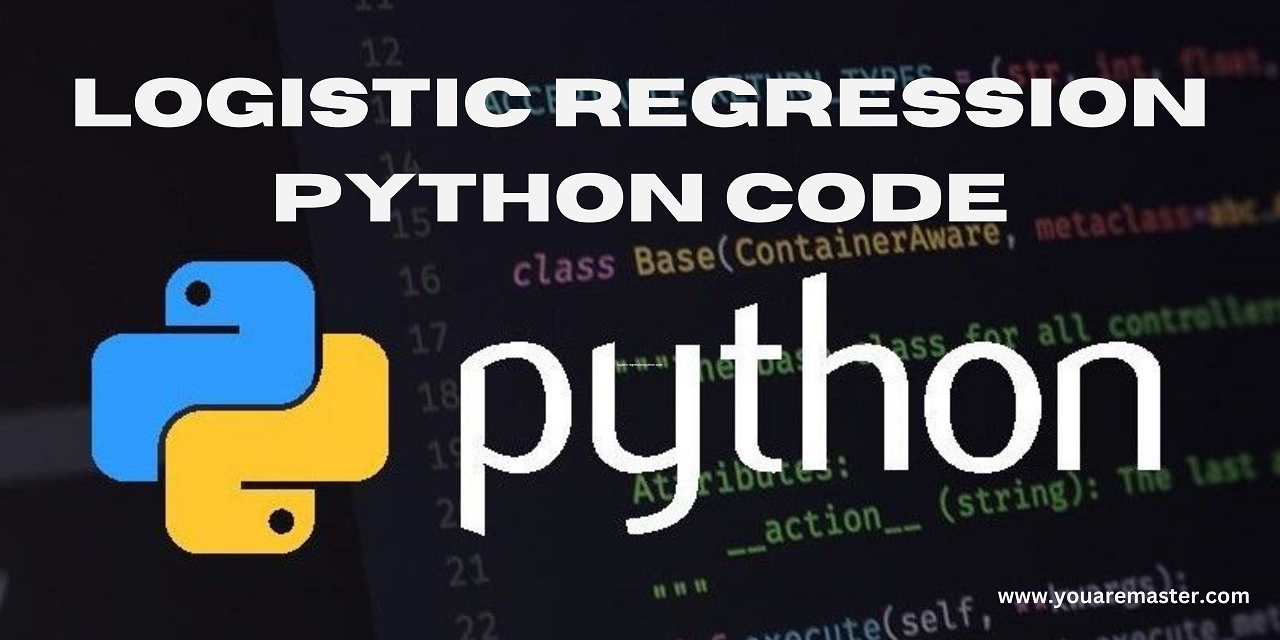
5. Example: Predicting Customer Churn with Logistic Regression
Dataset overview
Take a look at a dataset that includes client details and their churn status (churned or not).
Data preprocessing steps
To prepare the data for model training, clean it up, deal with missing values, and encode categorical variables.
Model building and evaluation
Develop a logistic regression model to forecast client attrition and assess the model’s effectiveness with pertinent indicators.
Certainly! Here is a straightforward Python logistic regression solution that makes use of the well-known Scikit-learn module. This code shows how to train and provide predictions for a logistic regression model using a synthetic dataset.
6. Logistic Regression Python Code
# Importing necessary libraries
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, classification_report
from sklearn.datasets import make_classification
# Generating synthetic dataset
X, y = make_classification(n_samples=1000, n_features=10, n_classes=2, random_state=42)
# Splitting the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initializing and training the logistic regression model
logistic_reg = LogisticRegression()
logistic_reg.fit(X_train, y_train)
# Making predictions on the testing set
y_pred = logistic_reg.predict(X_test)
# Calculating accuracy
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
# Generating classification report
print("\nClassification Report:")
print(classification_report(y_test, y_pred))
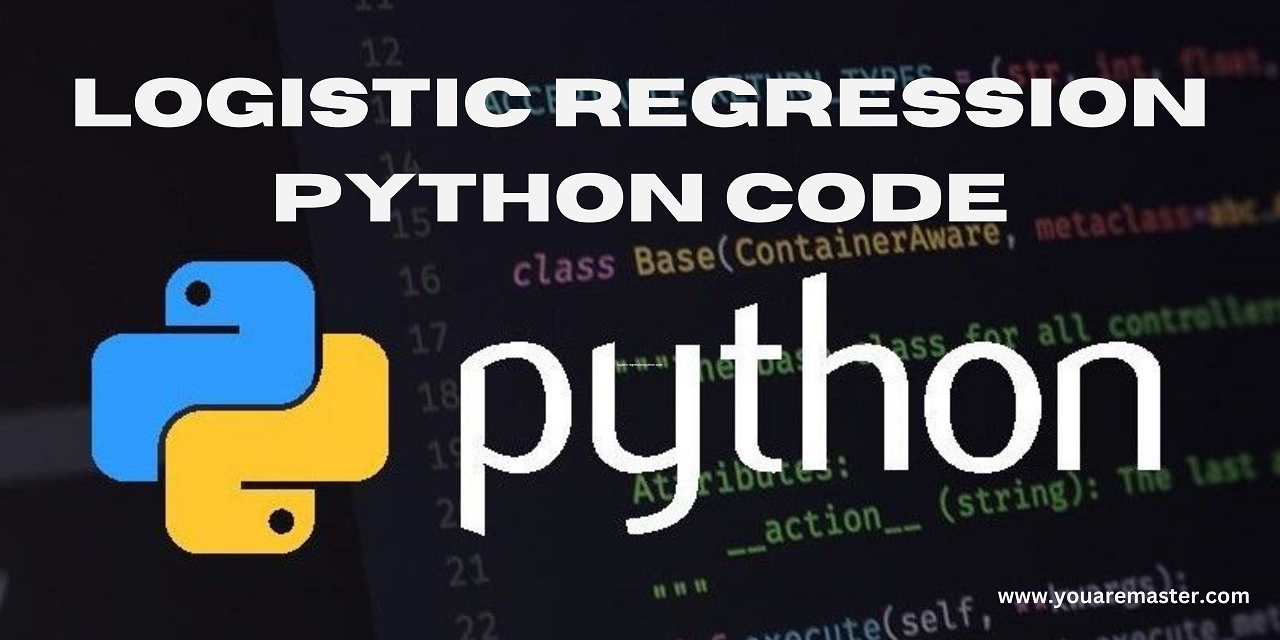
In above code:
- We first import the necessary libraries, including NumPy for numerical operations, Scikit-learn for machine learning algorithms, and specifically
make_classification
to generate a synthetic dataset. - We generate a synthetic dataset using
make_classification
, which creates a binary classification problem with 1000 samples, 10 features, and 2 classes. - Then, we split the dataset into training and testing sets
train_test_split
. - Next, we initialize a logistic regression model and train it on the training data
fit
. - After training, we make predictions on the testing set using
predict
. - We calculate the accuracy of the model using
accuracy_score
. - Finally, we print a classification report that includes precision, recall, F1-score, and support for each class.
Conclusion
For applications involving binary classification, logistic regression is a useful tool since it is straightforward, easy to understand, and effective. Data scientists may use logistic regression to efficiently solve problems in the real world and make well-informed judgements by grasping its ideas and putting them into practice in Python.
FAQs
What is logistic regression used for?
The main applications of logistic regression are in probability estimation and binary classification assignments.
How does logistic regression differ from linear regression?
Logistic regression predicts the probability of an instance belonging to a particular class, whereas linear regression predicts continuous values.
Can logistic regression handle multiclass classification?
As a binary classification algorithm, logistic regression is inherently binary, but extensions such as multinomial logistic regression can handle multiclass problems.
What are some common applications of logistic regression?
As a binary classification algorithm, logistic regression is inherently binary, but extensions such as sentiment analysis.
Is logistic regression suitable for handling imbalanced datasets?
In some cases, logistic regression can handle imbalanced datasets, but techniques like oversampling or undersampling may be necessary to achieve better results.