Mastering oops programming in python
A potent paradigm enabling developers to produce more flexible, modular, and reusable code is object-oriented programming (OOP). Beginning and experienced developers who wish to learn and use OOP ideas will find Python, renowned for its simplicity and readability, a great choice. The concepts of OOP in Python will be discussed in this blog article, together with how to use classes, objects, inheritance, polymorphism, and encapsulation to produce robust and maintainable code.
Introduction to OOP in Python
OOP is a programming paradigm that uses “objects,” which can contain data and code to manipulate it. Unlike procedural programming, OOP categorizes code into entities that combine state (data) and behavior (methods).
Python is popular for OOP due to its straightforward syntax, dynamic typing, and powerful built-in libraries. Its simplicity makes it excellent for developers who want to learn OOP without a high learning curve.
This article covers Python OOP basics, including class and object creation, inheritance, polymorphism, and encapsulation. By the end, you’ll know how to use these ideas to develop efficient Python code. Read MODULES AND PACKAGES IN PYTHON
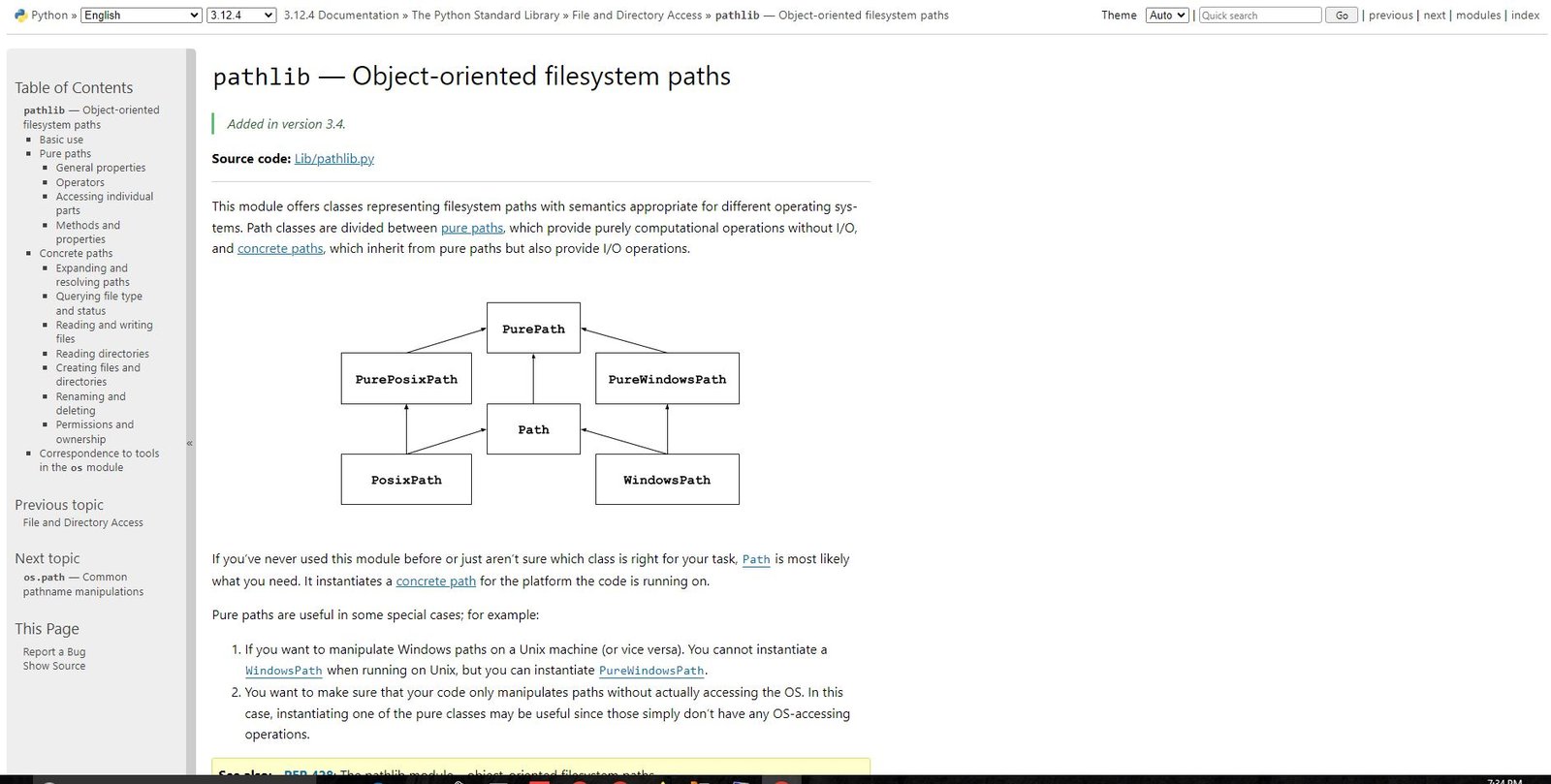
Python Classes and Objects
At the core of OOP are classes and objects. A class is a blueprint for creating objects (instances) and defining the properties and behaviors that the objects will have. An object is an instance of a class, representing a specific example of the blueprint.
To create a class in Python, use the `class` keyword followed by the class name. Here’s a simple example:
“`
class Dog:
def init(self, name, age):
self.name = name
self.age = age
def bark(self):
print(f”{self.name} says woof!”)
“`
In this example, `Dog` is a class with two properties (`name` and `age`) and one method (`bark`). To create an object from this class, call the class as if it were a function:
“`
my_dog = Dog(“Buddy”, 3)
my_dog.bark() # Output: Buddy says woof!
“`
This example illustrates how to define a class, initialize its properties with a constructor method (`init`), and create an instance (object) of that class.
Inheritance in Python
Inheritance allows a class (child class) to inherit attributes and methods from another class (parent class). This promotes code reuse and can lead to a more logical and hierarchical organization of classes.
To demonstrate inheritance, let’s extend our `Dog` class to create a `Poodle` class:
“`
class Poodle(Dog):
def init(self, name, age, color):
super().init(name, age)
self.color = color
def show_color(self):
print(f”{self.name} is {self.color}.”)
“`
Here, `Poodle` inherits from `Dog`, using the `super()` function to call the parent class’s `init` method and add property (`color`). Now, we can create a `Poodle` object and use both the inherited and new methods:
“`
my_poodle = Poodle(“Bella”, 2, “white”)
my_poodle.bark() # Output: Bella says woof!
my_poodle.show_color() # Output: Bella is white.
“`
This example showcases how inheritance can extend and specialize functionality in subclasses.
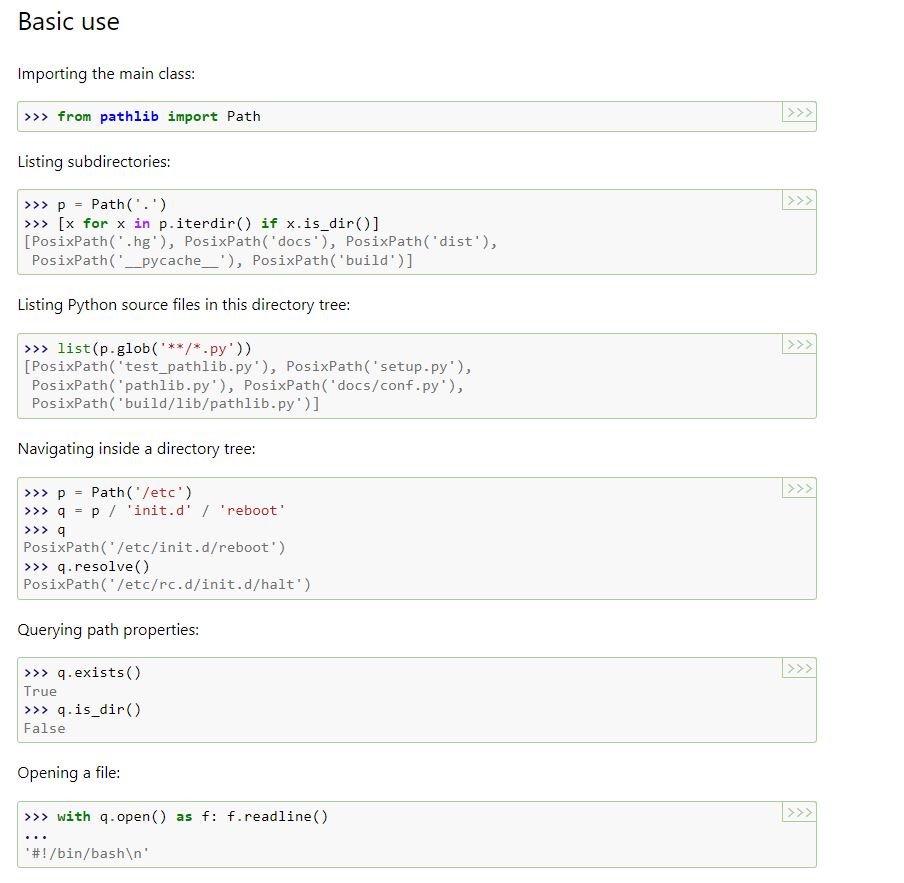
Polymorphism in Python
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables the same operation to behave differently in other courses.
Consider the following example with a `Cat` class in addition to our `Dog` class:
“`
class Cat:
def init(self, name, age):
self.name = name
self.age = age
def speak(self):
print(f”{self.name} says meow!”)
class Dog:
def init(self, name, age):
self.name = name
self.age = age
def speak(self):
print(f”{self.name} says woof!”)
“`
Both classes have a `speak` method but produce different outputs. Polymorphism allows us to call this method on objects of either class without knowing their specific type:
“`
def make_animal_speak(animal):
animal.speak()
my_cat = Cat(“Whiskers”, 4)
my_dog = Dog(“Buddy”, 3)
make_animal_speak(my_cat) # Output: Whiskers says meow!
make_animal_speak(my_dog) # Output: Buddy says woof!
“`
This example demonstrates how polymorphism can simplify code and enhance flexibility by allowing different types to be used interchangeably.
Encapsulation in Python
Encapsulation is the practice of hiding an object’s internal state and behavior and exposing only what is necessary. This is achieved through access modifiers like private and protected members.
In Python, encapsulation is implemented using underscores to indicate the intended visibility of attributes and methods:
“`
class BankAccount:
def init(self, owner, balance):
self.owner = owner
self.__balance = balance # Private attribute
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
else:
print(“Insufficient funds”)
def get_balance(self):
return self.__balance
“`
In this example, `__balance` is a private attribute that can only be accessed within the class through methods like `deposit`, `withdraw`, and `get_balance`. This ensures that the balance cannot be modified directly from outside the class, maintaining the integrity of the object’s state.
“`
account = BankAccount(“Alice”, 1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
account.__balance = 2000 # This will not change the balance
print(account.get_balance()) # Output: 1500
“`
Encapsulation helps maintain control over the data and prevents unintended interference with the object’s internal state.
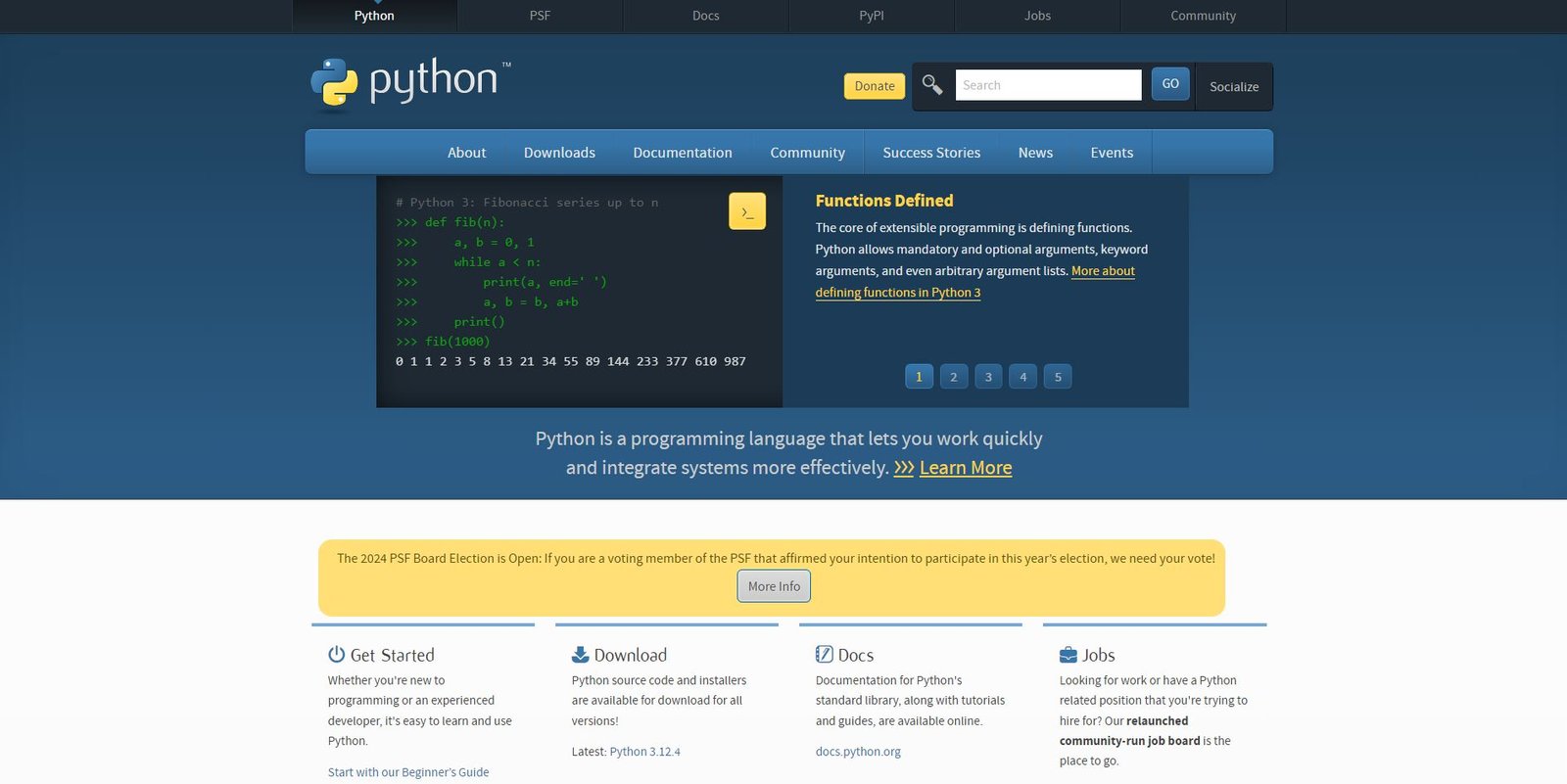
Practical Examples to Demonstrate OOP in Python
To solidify your understanding of OOP in Python, let’s explore some practical examples that demonstrate its principles in action. These examples will show you how to apply OOP concepts to solve real-world problems and create robust applications.
Example 1: Creating a Simple Inventory System
Let’s create a simple inventory system for a store that manages products and their quantities. We’ll use classes to model products and the inventory:
“`
class Product:
def init(self, name, price):
self.name = name
self.price = price
class Inventory:
def init(self):
self.products = {}
def add_product(self, product, quantity):
if product.name in self.products:
self.products[product.name] += quantity
else:
self.products[product.name] = quantity
def remove_product(self, product, quantity):
if product.name in self.products and self.products[product.name] >= quantity:
self.products[product.name] -= quantity
if self.products[product.name] == 0:
del self.products[product.name]
else:
raise ValueError(“Product not available or insufficient quantity”)
def get_inventory(self):
return self.products
“`
In this example, we define a `Product` class with attributes for name and price. The `Inventory` class manages a collection of products and provides methods to add and remove products and retrieve the current inventory.
Example 2 Building a Library Management System
Next, build a library management system that tracks books and borrowers. We’ll use classes to represent books, borrowers, and the library itself:
“`
class Book:
def init(self, title, author):
self.title = title
self.author = author
self.is_checked_out = False
def check_out(self):
if not self.is_checked_out:
self.is_checked_out = True
else:
raise ValueError(“Book already checked out”)
def return_book(self):
if self.is_checked_out:
self.is_checked_out = False
else:
raise ValueError(“Book not checked out”)
class Borrower:
def init(self, name):
self.name = name
self.borrowed_books = []
def borrow_book(self, book):
if not book.is_checked_out:
book.check_out()
self.borrowed_books.append(book)
else:
raise ValueError(“Book already checked out”)
def return_book(self, book):
if book in self.borrowed_books:
book.return_book()
self.borrowed_books.remove(book)
else:
raise ValueError(“Book not borrowed by this borrower”)
class Library:
def init(self):
self.books = []
self.borrowers = []
def add_book(self, book):
self.books.append(book)
def add_borrower(self, borrower):
self.borrowers.append(borrower)
def get_books(self):
return [book.title for book in self.books if not book.is_checked_out]
“`
In this example, we define `Book`, `Borrower`, and `Library` classes to represent the key elements of a library management system. The `Book` class includes methods to check out and return books, while the `Borrower` class manages a list of borrowed books. The `Library` class maintains a collection of books and borrowers and provides methods to add books and borrowers and retrieve available books.
Best Practices and Common Mistakes
While using OOP in Python, following best practices to ensure your code is clean, efficient, and maintainable is essential. Here are a few guidelines:
- Use Meaningful Names: Name your classes, methods, and attributes descriptively to make your code self-explanatory.
- Keep It Simple: Avoid overcomplicating your design. Use inheritance and polymorphism judiciously.
- Follow PEP 8: Adhere to Python’s style guide, PEP 8, to maintain code consistency and readability.
Common mistakes to avoid include:
- Overusing Inheritance: Inheritance is a powerful tool, but overusing it can lead to a complex and fragile codebase.
- Ignoring Encapsulation: Please encapsulate data correctly to avoid unexpected behaviors and bugs.
- Neglecting Documentation: Always document your classes and methods to provide clear guidance on their usage and purpose.
By following these best practices and avoiding common pitfalls, you can write better OOP code in Python.
Conclusion
Python object-oriented programming offers a strong means to produce maintainable, modular, reusable programs. Your Python programming abilities will be much enhanced by knowing and using the ideas of classes, objects, inheritance, polymorphism, and encapsulation.
All in all, we addressed:
OOP’s principles and the reasons Python is an intelligent choice.
How do we design and employ objects and classes?
Inheritance as a notion and its application.
Knowledge and application of polymorphism.
There is a need for encapsulation and Python’s methods to reach it.
Investigate and apply these ideas if you wish to improve your coding abilities and broaden your knowledge. Experiment with your tools and classes to find how you may apply OOP ideas to your projects. Joyful coding!
FAQ
Q: OOP is object-oriented programming.?
A: Object-oriented programming designs software with “objects”. Each object represents a real-world entity with data and manipulation methods.
Q Why should I use Python OOP?
A: Python’s readability and simplicity make it ideal for OOP. Python’s object-oriented support makes code modular, reusable, maintainable, and functional in larger projects.
Q: How can I start Python OOP?
A: Start with OOP’s essential building pieces, classes, and objects. Create simple classes, create objects, and try adding methods and attributes.
Q: What distinguishes classes from objects?
A: Classes define object behavior and properties. Objects are instances of classes, implementing their structure and behavior.
Q Python inheritance: what is it?
A: Inheritance lets classes inherit characteristics and methods from other classes. Code reuse can simplify complex hierarchies.
Please give an example of polymorphism.
Polymorphism lets objects of distinct classes behave like a superclass. For example, if the `Dog` and `Cat` classes inherit from `Animal`, calling `speak` on an `Animal` object will execute the relevant method for `Dog` or `Cat`.
Q: What is encapsulation, and why is it important?
A: Encapsulation protects an object’s data and exposes just relevant methods. Data access and modification must be controlled to reduce bugs and misuse.
Q Common OOP mistakes: How can we avoid them?
A: Use descriptive names, simplify your design, follow PEP 8 recommendations, avoid inheritance, encapsulate data, and describe your code.
Q: What resources can assist me in learning Python OOP?
A: Books like “Python Crash Course” by Eric Matthes, Coursera, edX, Codecademy, Stack Overflow, and GitHub can improve your Python OOP skills.