Master the Art of Define Python Functions
Understanding how to define Python functions is a fundamental skill for any developer. This guide will walk you through the process, providing clear examples and practical tips to help you master this essential programming concept. Whether you’re a beginner or an experienced coder, you’ll find valuable insights to enhance your coding skills.
Introduction to Python Functions
Functions are reusable blocks of code that perform specific tasks. They help make your code more organized, efficient, and manageable. In Python, functions are defined using the `def` keyword, which sets the stage for a block of code that can be executed whenever the function is called.
Python functions are versatile, supporting default arguments, keyword arguments, and variable-length arguments. These features make Python an excellent choice for both simple scripts and complex applications. Let’s explore how to define Python functions and make the most of their capabilities. Read MASTERING CONTROL FLOW IN PYTHON
Explaining the ‘def’ Keyword How to Define Python Functions
The `def` keyword is the foundation of Python functions. It signals the start of a function definition, followed by the function name and a pair of parentheses. Inside the parentheses, you can specify parameters that the function will accept.
To define a basic function, use the following syntax:
“`
def function_name(parameters):
# Function body
# Your code here
return value
“`
This structure is easy to understand and implement. The function name should be descriptive, clearly indicating what the function does. Parameters are optional and can vary based on the function’s requirements.
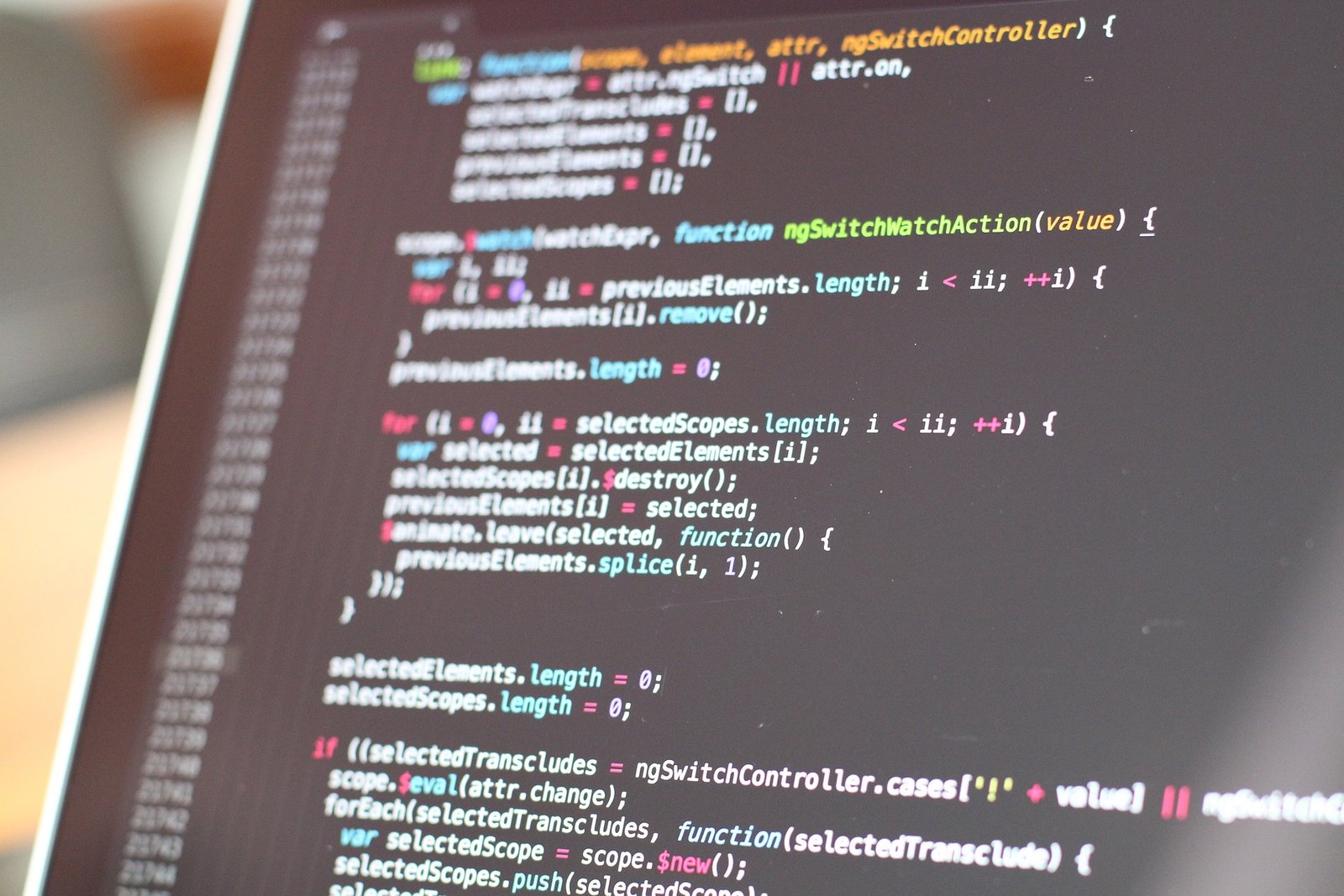
The Anatomy of a Python Function Parameters and Return Values
A typical Python function consists of three main parts:
- Function name: A descriptive name that identifies the function.
- Parameters (optional): Variables passed to the function to customize its behavior.
- Return value (optional): The result that the function produces.
Here’s an example of a simple function with parameters and a return value:
“`
def add_numbers(a, b):
result = a + b
return result
“`
In this example, `add_numbers` is the function name, `a` and `b` are parameters, and `result` is the return value. When the function is called with specific arguments, it adds the numbers and returns the sum.
Working with Default Arguments and Keyword Arguments
Default arguments allow you to specify default values for parameters. If no argument is provided for a parameter with a default value, the function uses the default value. This feature makes functions more flexible and easier to use.
Here’s an example:
“`
def greet(name, greeting=”Hello”):
return f”{greeting}, {name}!”
“`
Keyword arguments enable you to call functions with arguments in any order. You specify the argument name and value, making the code more readable and reducing the chance of errors.
Example:
“`
print(greet(name=”Alice”, greeting=”Hi”))
print(greet(greeting=”Hey”, name=”Bob”))
“`
Both calls produce the same result, demonstrating the flexibility of keyword arguments.
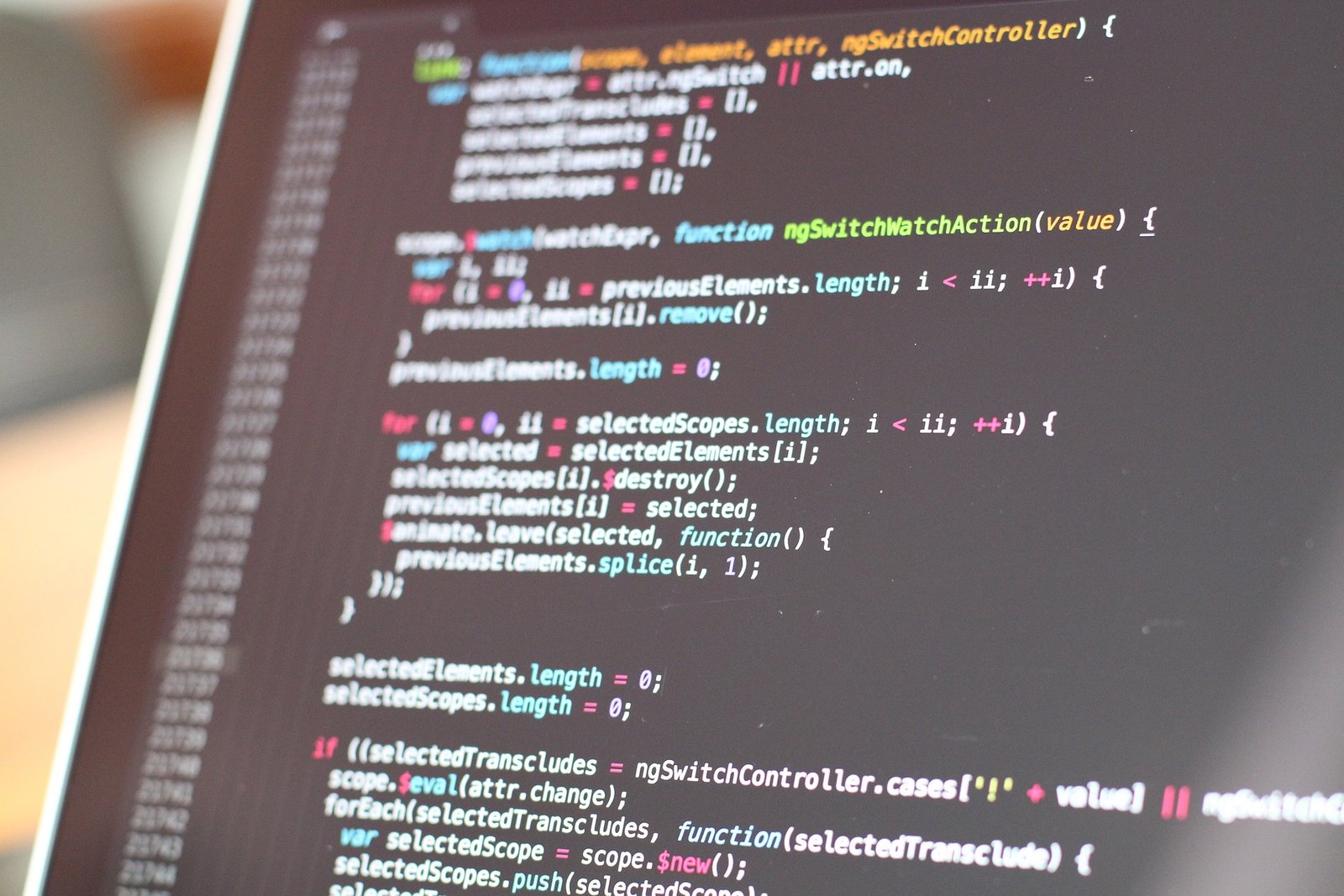
Understanding Variable-Length Arguments in Python Functions
Variable-length arguments allow functions to accept an arbitrary number of arguments. This feature is useful when you don’t know in advance how many arguments the function will receive.
There are two types of variable-length arguments:
- `*args`: For non-keyword arguments.
- `kwargs`**: For keyword arguments.
Example of using `*args`:
“`
def sum_numbers(*args):
return sum(args)
“`
Example of using `**kwargs`:
“`
def display_info(**kwargs):
for key, value in kwargs.items():
print(f”{key}: {value}”)
“`
These features provide great flexibility, allowing you to create functions that handle a wide range of inputs.
Common Mistakes and Best Practices in Defining Python Functions
When defining Python functions, it’s essential to follow best practices to avoid common pitfalls. Here are some tips to keep in mind:
- Choose descriptive names: Function names should clearly describe their purpose.
- Keep functions short and focused: Each function should perform a single task.
- Use comments and docstrings: Document your functions to explain their behavior and usage.
Common mistakes include:
- Misplaced return statements: Ensure the return statement is inside the function body.
- Incorrect indentation: Python relies on indentation to define blocks of code, so be consistent.
- Overusing global variables: Avoid using global variables inside functions, as they can lead to unexpected behaviors.
Real-World Examples and Use Cases
Understanding how to define Python functions is crucial, but seeing them in action can provide additional clarity. Here are some real-world examples and use cases:
- Data processing:
“`
def process_data(data):
cleaned_data = [x.strip() for x in data if x]
return cleaned_data
“`
- Web development:
“`
def render_template(template_name, **context):
template = load_template(template_name)
return template.render(context)
“`
- Automation:
“`
def send_email(to, subject, body):
email = EmailMessage()
email[‘To’] = to
email[‘Subject’] = subject
email.set_content(body)
smtp.send_message(email)
“`
These examples demonstrate how Python functions can simplify complex tasks and improve code maintainability.
Conclusion
Mastering the art of defining Python functions is essential for any developer. Functions make your code more organized, reusable, and easier to understand. By following best practices and leveraging features like default arguments, keyword arguments, and variable-length arguments, you can create powerful and flexible functions that enhance your programming skills.
If you’re ready to take your coding skills to the next level, start experimenting with Python functions today. The more you practice, the more proficient you’ll become. Happy coding!
Frequently Asked Questions (FAQ)
Q: What is the purpose of a function in Python?
A: Functions are used to encapsulate code into reusable blocks, making your programs more organized and easier to maintain. They enable you to break down complex problems into smaller, manageable pieces.
Q: How do I call a function in Python?
A: You call a function by specifying its name followed by parentheses. If the function requires arguments, you include them within the parentheses. For example, `add_numbers(2, 3)` calls the `add_numbers` function with 2 and 3 as arguments.
Q: Can a function return multiple values?
A: Yes, a function can return multiple values by using tuples. For instance:
“`
def get_coordinates():
return (10, 20)
“`
You can then unpack these values when calling the function: `x, y = get_coordinates()`.
Q: What are lambda functions and when should I use them?
A: Lambda functions are anonymous, single-expression functions declared using the `lambda` keyword. They are useful for short, throwaway functions that you don’t need to reuse. Example:
“`
square = lambda x: x * x
print(square(5)) # Outputs: 25
“`
Q: How can I document my Python functions effectively?
A: Use docstrings to document your functions. Docstrings are string literals that appear as the first statement in a function. They provide a convenient way to associate documentation with functions. Example:
“`
def add_numbers(a, b):
“””
Add two numbers and return the result.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
“””
return a + b
“`
Q: What is the difference between `*args` and `kwargs`?**
A: `*args` allows you to pass a variable number of non-keyword arguments to a function. `**kwargs` allows you to pass a variable number of keyword arguments. This provides flexibility in the number of inputs your function can handle.
Q: Why should I avoid using global variables in functions?
A: Using global variables can lead to code that is difficult to debug and maintain. It can also cause unexpected behaviors as the global state can be modified from different parts of the program. Instead, pass variables as arguments to your functions.
Understanding these fundamental concepts will greatly enhance your ability to write efficient and robust Python code.