Youtube Thumbnail Downloader
Integrating HTML Code into a Django Project: A Step-by-Step Guide
Table of Contents
-
Introduction
-
Create a Django Project
-
Create a Django App
-
Replace Contents in
views.py
-
Configure URL Routing
-
Create the
templates
folder -
Directory Structure
-
Run the Django Development Server
-
Visit the YouTube Thumbnail Downloader Page
-
Configuring Django Settings
-
Customization and Additional Features
-
Troubleshooting Tips
-
Best Practices for Django Project Structure
-
Conclusion
-
FAQs
Introduction
Django, a high-level Python web framework, makes integrating HTML code into a project a breeze. In this guide, we’ll walk through the steps to seamlessly incorporate provided HTML code into a Django project, specifically for a YouTube Thumbnail Downloader. Let’s dive in!
1. Create a Django Project
django-admin startproject YouTubeThumbnailDownloader cd YouTubeThumbnailDownloader
2. Create a Django App
python manage.py startapp thumbnail_downloader
3. Replace Contents in views.py
Replace the contents of thumbnail_downloader/views.py
with the provided code:
from django.shortcuts import render def youtube_thumbnail_downloader(request): return render(request, 'thumbnail_downloader/youtube_thumbnail_downloader.html')
4. Configure URL Routing
Modify the urls.py
in the thumbnail_downloader
app:
from django.urls import path from .views import youtube_thumbnail_downloader urlpatterns = [ path('youtube-thumbnail-downloader/', youtube_thumbnail_downloader, name='youtube_thumbnail_downloader'), ]
Include these app-specific URLs in the project’s urls.py
:
from django.contrib import admin from django.urls import include, path urlpatterns = [ path('admin/', admin.site.urls), path('', include('thumbnail_downloader.urls')), ]
5. Create the templates
folder
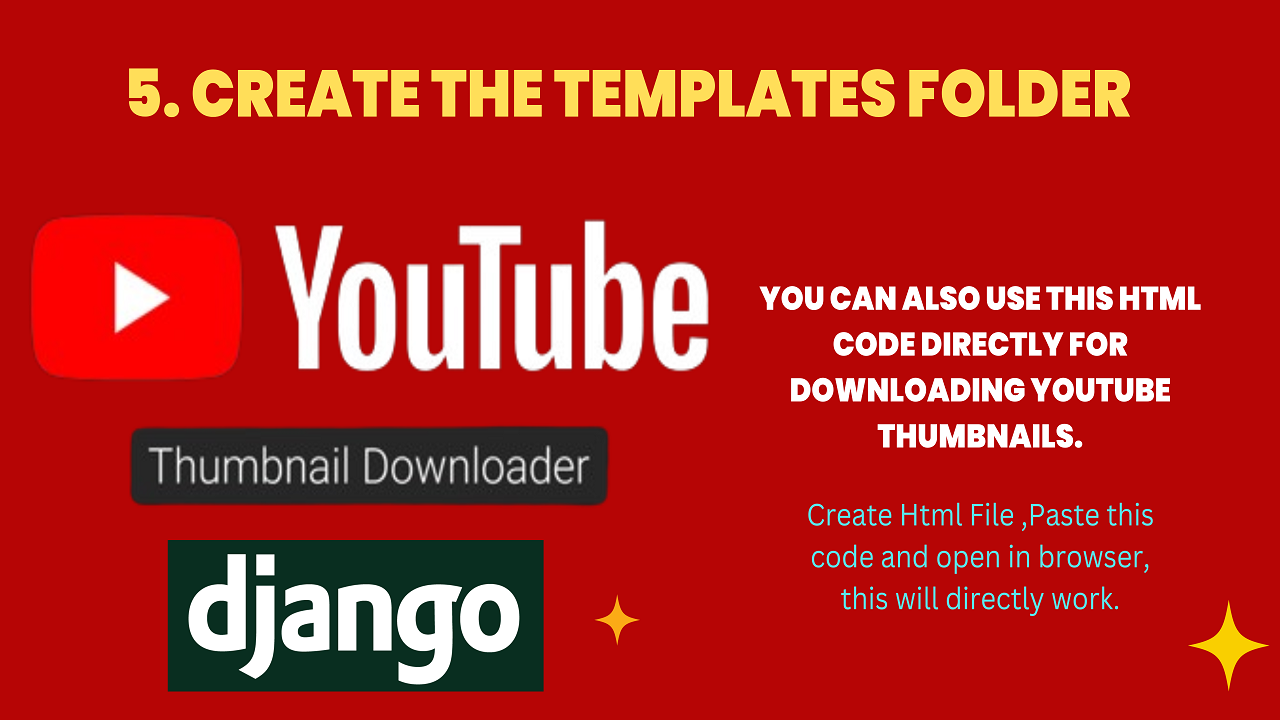
You can also use this HTML code directly for downloading Youtube thumbnails.
Create Html File ,Paste this code and open in browser, this will directly work.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>YouTube Thumbnail Downloader</title> <style> body { font-family: Arial, sans-serif; text-align: center; margin: 20px; } #thumbnailContainer { margin-top: 20px; } .thumbnail { max-width: 100%; margin-top: 10px; } </style> </head> <body> <h1>YouTube Thumbnail Downloader</h1> <label for="videoUrl">Enter YouTube Video URL:</label> <input type="text" id="videoUrl" placeholder="https://www.youtube.com/watch?v=VIDEO_ID" /> <button onclick="downloadThumbnails()">Download Thumbnails</button> <div id="thumbnailContainer"> <!-- Thumbnails will be displayed here --> </div> <script> function downloadThumbnails() { const videoUrl = document.getElementById('videoUrl').value; const videoId = getVideoId(videoUrl); if (videoId) { const mediumThumbnailUrl = `https://img.youtube.com/vi/${videoId}/mqdefault.jpg`; const fullThumbnailUrl = `https://img.youtube.com/vi/${videoId}/maxresdefault.jpg`; // Display the thumbnails displayThumbnail('Medium Size', mediumThumbnailUrl); displayThumbnail('Full Size', fullThumbnailUrl); } else { alert('Invalid YouTube video URL. Please enter a valid URL.'); } } function displayThumbnail(size, url) { const thumbnailContainer = document.getElementById('thumbnailContainer'); thumbnailContainer.innerHTML += ` <p>${size}:</p> <img class="thumbnail" src="${url}" alt="${size} Thumbnail" /> <button onclick="downloadThumbnail('${url}', '${size}')">Download ${size} Thumbnail</button> <br> `; } function downloadThumbnail(url, size) { // For simplicity, let's open the image in a new tab for download window.open(url, `_blank`).focus(); } function getVideoId(url) { const regex = /(?:https?:\/\/)?(?:www\.)?(?:youtube\.com\/(?:[^\/\n\s]+\/\S+\/|(?:v|e(?:mbed)?)\/|\S*?[?&]v=)|youtu\.be\/)([a-zA-Z0-9_-]{11})/; const match = url.match(regex); return match ? match[1] : null; } </script> </body> </html>
6. Directory Structure
YouTubeThumbnailDownloader/ │ ├── YouTubeThumbnailDownloader/ │ ├── settings.py │ ├── urls.py │ └── wsgi.py │ └── thumbnail_downloader/ ├── migrations/ ├── templates/ │ └── thumbnail_downloader/ │ └── youtube_thumbnail_downloader.html ├── __init__.py ├── admin.py ├── apps.py ├── models.py ├── tests.py └── views.py
7. Run the Django Development Server
python manage.py runserver
8. Visit the YouTube Thumbnail Downloader Page
Visit http://127.0.0.1:8000/youtube-thumbnail-downloader/
to see the YouTube Thumbnail Downloader page.
9. Configuring Django Settings
Adjust Django settings like INSTALLED_APPS
and TEMPLATES
based on your project’s requirements.
10. Customization and Additional Features
Feel free to customize the project further and add additional features to meet your specific needs.
11. Troubleshooting Tips
If you encounter issues, refer to Django’s documentation or community forums for troubleshooting tips.
12. Best Practices for Django Project Structure
Adopt best practices for maintaining a clean and efficient Django project structure.
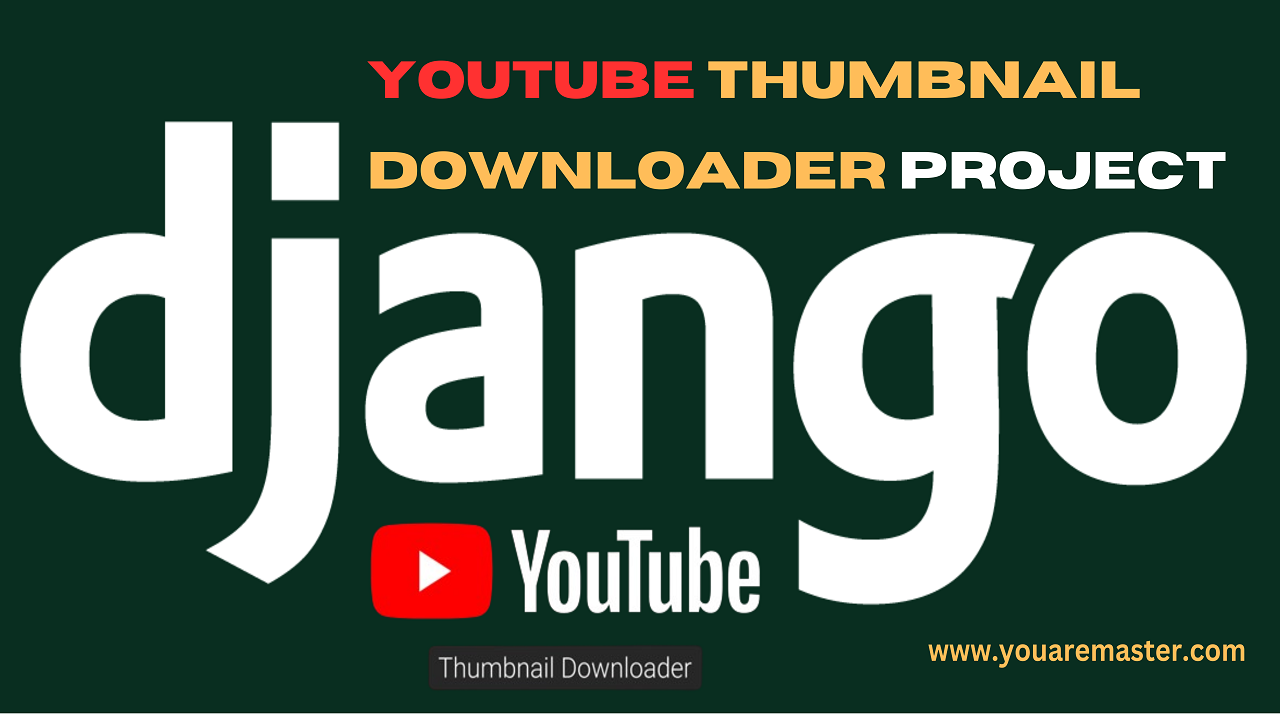
13. Conclusion
Integrating HTML code into a Django project is a straightforward process. By following these steps, you’ve successfully set up a YouTube Thumbnail Downloader page. Customize, explore, and enjoy the flexibility of Django!
14. FAQs
- Is Django suitable for small projects?
- Absolutely! Django’s versatility makes it suitable for projects of all sizes.
- How can I add CSS styles to my Django project?
- Create a
static
folder, add your CSS files, and link them in your HTML templates.
- Create a
- Can I integrate JavaScript with Django?
- Yes, you can include JavaScript in your Django project for dynamic functionality.
- What is the purpose of the
migrations
folder?- The
migrations
folder handles database schema changes over time.
- The
- Are there Django hosting recommendations?
- Popular platforms like Heroku, AWS, and DigitalOcean are great for Django hosting.
Certainly! Integrating HTML code into a Django project offers several benefits:
- Structured Web Development:
- Django provides a structured framework, ensuring a clear separation of concerns. HTML code integration becomes part of a well-organized project structure.
- Rapid Development:
- Django’s high-level abstractions and built-in features facilitate rapid development. Developers can focus on integrating HTML and building functionality rather than dealing with low-level details.
- Template Engine:
- Django’s template engine simplifies the integration of HTML by allowing the inclusion of dynamic content and logic directly in HTML files. This enhances code readability and maintainability.
- Modularity and Reusability:
- By breaking the project into apps and components, developers can create modular and reusable HTML templates. This promotes a cleaner codebase and makes it easier to scale the project.
- ORM (Object-Relational Mapping):
- Django’s ORM system abstracts database interactions, reducing the need for manual SQL queries. This separation of concerns ensures that HTML integration is primarily focused on presentation rather than database handling.
- Built-in Security Measures:
- Django incorporates built-in security features, such as protection against common web vulnerabilities. This ensures that HTML integration follows best security practices.
- Community and Documentation:
- Django has a robust community and extensive documentation. Developers benefit from a wealth of resources, including tutorials, forums, and third-party packages, making the integration of HTML code more accessible.
- Scalability:
- Django’s scalability features, such as middleware and efficient database handling, ensure that projects can grow seamlessly. HTML integration is part of a scalable architecture.
- Consistent URL Routing:
- Django’s URL routing system provides a consistent and clean way to map URLs to views. This makes it straightforward to link HTML templates to specific views within the project.
- Versatility in Frontend Technologies:
- Django is frontend-agnostic, allowing developers to use various frontend technologies alongside HTML, such as JavaScript frameworks (e.g., React, Vue) and CSS preprocessors. This flexibility enables modern and dynamic user interfaces.
- Testing and Debugging Tools:
- Django includes tools for testing and debugging, ensuring that integrated HTML code can be thoroughly tested for functionality and performance.
- Community Packages and Extensions:
- The Django ecosystem offers numerous community-contributed packages and extensions that can enhance HTML integration, providing additional features and functionalities.
In summary, integrating HTML into a Django project combines the flexibility of HTML with the robust features and structure provided by the Django framework, resulting in efficient and scalable web development.
Note:
This guide serves as a reference for developers aiming to seamlessly integrate HTML into Django projects, providing both practical steps and additional insights into customization and troubleshooting.