How To Make A Chat App In Django – 2024
Introduction
Embark on a journey to create a dynamic chat app in Django, an open-source web framework for Python. This guide ensures a smooth ride from the basics to advanced features, making the process accessible for all.
Setting the Stage
Understanding the significance of chat apps in today’s digital landscape lays the foundation for our Django venture. Discover why Django is the perfect choice for such a dynamic project.
Prerequisites
Before diving into Django, ensure you have a solid grasp of Python. Walk through the installation of Django, setting the stage for an efficient development process.
Project Initialization
Creating a Django project is the first step. We’ll delve into project structure, configuration, and the creation of Django apps to organize your work effectively.
Designing the Database
Explore the intricacies of database design in Django. Understand models, relationships, and make informed choices between SQLite and other databases.
User Authentication
Security is paramount. Learn to implement user accounts securely, fortifying your chat app against unauthorized access.
Building the Chat Interface
User experience is key. Master the art of front-end development in Django, ensuring an intuitive and visually appealing chat interface.
Integrating WebSocket
Unlock real-time communication by integrating WebSocket. We’ll guide you through the setup and implementation using Django Channels.
Implementing Chat Functionality
Dive deep into handling messages, notifications, and ensuring a seamless chat experience for your users.
Testing the App
Quality assurance matters. Learn the ropes of unit and integration testing, coupled with effective debugging techniques.
Deployment Options
Ready to share your creation with the world? Explore hosting services and scaling strategies to deploy your Django chat app.
Optimization Techniques
Fine-tune your app for optimal performance. Explore performance tweaks and database optimization strategies.
Security Measures
Safeguard user data with SSL implementation and robust security measures, ensuring a secure environment.
User Feedback
User satisfaction is our goal. Incorporate user suggestions and conduct beta testing for valuable insights.
Future Enhancements
Dream big! Explore the possibilities of AI integration and additional features to take your chat app to the next level.
Troubleshooting Common Issues
Navigate through common challenges with our troubleshooting tips and tap into the supportive Django community.

Step 1: Set up your Django project
django-admin startproject mychat cd mychat
Step 2: Create a Django app
python manage.py startapp chat
Step 3: Install required packages
pip install channels
Step 4: Update project settings
mychat/settings.py
INSTALLED_APPS = [ # ... 'channels', 'chat', ] # ... # Add this at the end of the file ASGI_APPLICATION = 'mychat.asgi.application'
Step 5: Create a consumer for handling WebSocket connections
chat/consumers.py
import json from channels.generic.websocket import AsyncWebsocketConsumer class ChatConsumer(AsyncWebsocketConsumer): async def connect(self): self.room_name = self.scope['url_route']['kwargs']['room_name'] self.room_group_name = f"chat_{self.room_name}" # Join room group await self.channel_layer.group_add( self.room_group_name, self.channel_name ) await self.accept() async def disconnect(self, close_code): # Leave room group await self.channel_layer.group_discard( self.room_group_name, self.channel_name ) async def receive(self, text_data): text_data_json = json.loads(text_data) message = text_data_json['message'] # Send message to room group await self.channel_layer.group_send( self.room_group_name, { 'type': 'chat.message', 'message': message } ) async def chat_message(self, event): message = event['message'] # Send message to WebSocket await self.send(text_data=json.dumps({ 'message': message }))
Step 6: Create a routing configuration
chat/routing.py
from django.urls import re_path from . import consumers websocket_urlpatterns = [ re_path(r'ws/chat/(?P<room_name>\w+)/$', consumers.ChatConsumer.as_asgi()), ]
Step 7: Create an ASGI application
mychat/asgi.py
import os from django.core.asgi import get_asgi_application from channels.routing import ProtocolTypeRouter, URLRouter from channels.auth import AuthMiddlewareStack from chat.routing import websocket_urlpatterns os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'mychat.settings') application = ProtocolTypeRouter({ "http": get_asgi_application(), "websocket": AuthMiddlewareStack( URLRouter( websocket_urlpatterns ) ), })
Step 8: Create a simple HTML template for the chat
<!DOCTYPE html> <html> <head> <title>Chat Room</title> </head> <body> <div id="chat"> <input id="messageInput" type="text" placeholder="Type your message..."> <button onclick="sendMessage()">Send</button> <ul id="chatMessages"></ul> </div> <script> const roomName = "{{ room_name }}"; const chatSocket = new WebSocket(`ws://${window.location.host}/ws/chat/${roomName}/`); chatSocket.onmessage = function (e) { const data = JSON.parse(e.data); const message = data.message; const chatMessages = document.getElementById('chatMessages'); const messageElement = document.createElement('li'); messageElement.innerText = message; chatMessages.appendChild(messageElement); }; function sendMessage() { const messageInput = document.getElementById('messageInput'); const message = messageInput.value; chatSocket.send(JSON.stringify({'message': message})); messageInput.value = ''; } </script> </body> </html>
Step 9: Update project URLs
mychat/urls.py
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('chat/', include('chat.urls')), ]
Step 10: Create chat app URLs
chat/urls.py
from django.urls import re_path from . import consumers websocket_urlpatterns = [ re_path(r'ws/chat/(?P<room_name>\w+)/$', consumers.ChatConsumer.as_asgi()), ] urlpatterns = [ # You can add views for rendering chat interfaces here if needed ] # Add WebSocket URL patterns application = ProtocolTypeRouter({ "http": get_asgi_application(), "websocket": AuthMiddlewareStack( URLRouter( websocket_urlpatterns ) ), })
Step 11: Run migrations and start the development server
python manage.py makemigrations python manage.py migrate python manage.py runserver
Step 12: Test the WebSocket connection
Open your web browser and visit http://127.0.0.1:8000/chat/room_name/
. Open the browser console and run the following JavaScript code:
const socket = new WebSocket('ws://127.0.0.1:8000/ws/chat/room_name/'); socket.onmessage = function (e) { console.log(e.data); }; socket.onopen = function (e) { socket.send(JSON.stringify({'message': 'Hello, World!'})); };
Now, you can visit http://127.0.0.1:8000/chat/room_name/
in your browser, and you should see a simple chat interface. Open multiple tabs to simulate different users and see real-time chat updates.
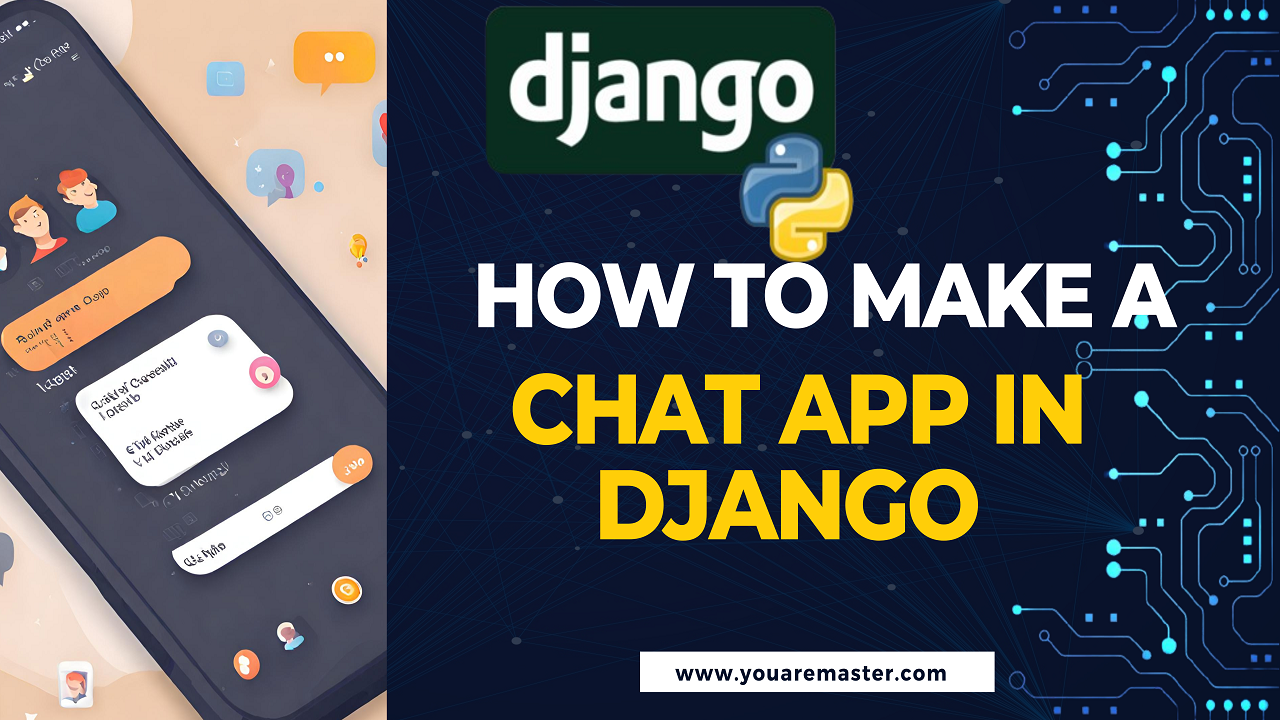
FAQs
Q: Is Django suitable for beginners?
A: Absolutely! Django’s user-friendly structure makes it accessible for developers of all levels.
Q: How can I add real-time features to my chat app?
A: By integrating WebSocket through Django Channels, enabling seamless real-time communication.
Q: What hosting service is recommended for Django apps?
A: Popular choices include Heroku, AWS, and DigitalOcean. Select based on your project’s needs.
Q: Can I customize the chat interface’s appearance?
A: Certainly! Django offers flexibility in front-end development for a personalized user experience.
Q: Are there any security risks with Django?
A: Security is a priority in Django. Follow best practices, use SSL, and stay updated to mitigate risks.
Q: How can I contribute to the Django community?
A: Engage in forums, contribute to open-source projects, and attend Django events to be an active part of the community.
Conclusion
A hearty congratulations is in order! You’ve mastered the complex realm of Django chat app development and created a lively environment that encourages easy communication. Let’s take a time to review the essential stages, stress the value of maintaining curiosity, and delve into the plethora of opportunities that await in the ever-evolving field of web development as we come to the end of this extensive tutorial.
Recap of Key Steps
The journey began with a solid foundation—understanding the significance of chat apps in the digital landscape and recognizing Django as the optimal framework for such a project. From there, we delved into prerequisites, ensuring a strong Python foundation and setting the stage for a smooth development process.
Project initialization was the next crucial step, where we created a Django project and app, establishing an organized structure for our work. Designing the database followed, shedding light on models, relationships, and the critical decision-making process between SQLite and other databases.
User authentication took center stage to fortify our chat app against unauthorized access. Building the chat interface ensured a user-friendly experience, and integrating WebSocket elevated our app to real-time communication heights. Implementing chat functionality, testing the app, and exploring deployment options were subsequent steps, preparing our creation for the digital stage.
Optimization techniques and security measures added finesse to the final product, ensuring optimal performance and safeguarding user data. User feedback, future enhancements, and troubleshooting common issues rounded off our development journey, leaving us with a robust Django chat app ready for deployment.
Stay Curious
Curiosity is the driving force behind innovation. As a developer, maintaining a curious mindset is paramount. The world of web development is ever-evolving, and technologies continuously advance. Stay curious about emerging trends, frameworks, and tools that could enhance your chat app or inspire your next project.
Django itself is a versatile framework, and its community is vibrant. Explore the latest updates, engage in forums, and contribute to open-source projects. The more you immerse yourself in the community, the more you’ll learn and grow as a developer.
Explore Further Possibilities
After one job is over, a plethora of opportunities arise. With the information and experience you’ve got from creating a Django chat app, think about looking into other options. There are still things to learn about and explore, such integrating artificial intelligence (AI), adding functionality, and experimenting with cutting-edge technology.
Consider the potential development of your chat app. Could chatbots powered by AI help it? Are there any features that consumers have been requesting that might help your software advance? The opportunities are only limited by your creativity, but with the talents you’ve developed along the way, you can make those ideas a reality.
Embracing the Dynamic World of Web Development
Being a part of the dynamic field of web development requires embracing change. The adventure doesn’t stop here, regardless of experience level—it continues to grow. Continue to hone your abilities, see obstacles as opportunities, and let the dynamic nature of web development to help you advance.
In summary, developing a Django chat app involves more than simply writing code; it also entails building a platform that encourages deep communication. As you go, never forget that every keystroke, every solved problem, and every creative idea adds to the dynamic fabric of web development.
Once again, congratulations on your accomplishment. With every line of code you create, the world of web development will open up new possibilities for you if you maintain your curiosity and keep writing.